HTML5 web based video player component from Smarter AI
Authentication
The Smarter AI uses a Tenant-based user authentication system. You need a tenant access token to access any Camera or Event for the Video Player. There are two steps to obtain tenant access.
1. User Login
To obtain a token, make a POST request to https://api.anyconnect.com/v5/auth/login with the following request body:
{
"authenticationType": "PASSWORD",
"email": "<YOUR USERNAME HERE>",
"password": "<YOUR PASSWORD HERE>"
}
You may receive a response like this:
{
"accountId": "90d****f75",
"email": "*****",
"refreshToken": "eyJhbGciO****nYv4ZXNvg",
"tenantAccessList": [
{
"tenantId": "****",
"tenantName": "Smarter AI Development",
"userRole": "END-USER"
}
//...
]
}
The field refreshToken
is used for the next step during Tenant Login.
2. Tenant Login
In the login API response, you'll get refreshToken and a list of tenants at tenantAccessList.
refreshToken
– Token form login API response refreshTokentenantId
– Pick one of the tenants from the tenantAccessList
You can now make a POST request to https://api.anyconnect.com/v5/auth/tenant-access with the following request body:
{
"email": "<YOUR EMAIL HERE>",
"tenantId": "<YOUR TENANT ID>",
"token": "<REFRESH TOKEN FROM LOGIN API>"
}
You may receive a response like this:
{
"accessToken": "eyJhbGciOiJSUzI1NiJ9*****g",
"accessTokenExpiry": 16894*****9822,
"refreshToken": "eyJhbGciOiJSUzI1NiJ9.******R4pg",
"refreshTokenExpiry": 16*****769822,
"userId": 3977*****33590,
"sessionId": "efd65******a1e3befd6",
"tenantId": "e218aacc-*****-e5966b1722dc"
}
The following information from this response is required:
refreshToken
– This will be our refresh-token for the dashcam-player componentaccessToken
– This will be our access-token for the dashcam-player component
Video Player Integration
Add the following imports for using the dashcam-player:
<!-- Imports for Dashcam Player -->
<link href="https://storage.googleapis.com/sai-web-libs/dashcam-player/2025.04.02/dashcam-player.min.css" rel="stylesheet" />
<script defer="defer" src="https://storage.googleapis.com/sai-web-libs/dashcam-player/2025.04.02/dashcam-player.min.js"></script>
Sample code for different types of playback:
<dashcam-player
tenant-id="<TENANT ID>"
refresh-token="<REFRESH TOKEN FROM TENANT ACCESS API>"
access-token="<ACCESS TOKEN FROM TENANT ACCESS API>"
event-id="<THE EVENT ID>"
hide-header
style="width: 100vw; height: 100vh"
/>
<dashcam-player
tenant-id="<TENANT ID>"
refresh-token="<REFRESH TOKEN FROM TENANT ACCESS API>"
access-token="<ACCESS TOKEN FROM TENANT ACCESS API>"
endpoint-id="<THE ENDPOINT ID OF THE CAMERA>"
hide-header
hide-widgets
hide-map
style="width: 100vw; height: 100vh"
/>
<dashcam-player
tenant-id="<TENANT ID>"
refresh-token="<REFRESH TOKEN FROM TENANT ACCESS API>"
access-token="<ACCESS TOKEN FROM TENANT ACCESS API>"
endpoint-id="<THE ENDPOINT ID OF THE CAMERA>"
time="<THE START TIMESTAMP (UNIX)>"
hide-header
style="width: 100vw; height: 100vh"
/>
Video Player Attributes
Properties | Description |
---|---|
tenant-id | The Tenant ID. |
refresh-token | The refresh token from the Tenant Login. |
access-token | The access token from the Tenant Login. |
event-id | The ID of the Event for Event playback. |
endpoint-id | The Endpoint ID of the Camera for Live or Recording playback. |
time | A UNIX timestamp to start the Recording playback. |
hide-widgets | To hide the Player Widgets. |
hide-map | To hide the Player Map. |
hide-header | To hide the information bar containing the Camera name, Trigger name, etc. |
hide-controls | To hide the player controls. |
hide-time-browser | To hide the day-hour-based timeline browser. |
hide-bounding-boxes | To hide the bounding boxes |
disable-auto-wake | To disable automatic waking up from the parking mode |
hide-speed-widget | To hide the speed widget from the video |
show-event-list | To display a list of related events beside the player |
stacked-view | To configure the video player to display imagers in stack view mode. |
force-thumbnail-view | To configure the video player to display imagers in thumbnail view mode. |
force-split-view | To configure the video player to display imagers in split view mode. |
Attributes for different types of playback
Action | tenant-id | token | eventId | endpoint-id | time |
---|---|---|---|---|---|
Live Streaming | Required | Required | Required | Exclude | Exclude |
Event Playback | Required | Required | Exclude | Required | Exclude |
Recording Playback | Required | Required | Required | Exclude | Required |
Video Player Interaction
You can interact with the component by calling methods or using getters and setters. An example:
const player = document.querySelector('dashcam-player');
player.addEventListener('load', () => {
player.play(); // to start the playback
player.pause(); // to pause the playback
player.rewind(); // jump to start during event playback
player.jumpForward(); // jump forward one unit
player.jumpBackward(); // jump backward one unit
console.log(player.currentTime); // get the current timestamp
console.log(player.playbackSpeed); // get the playback speed
player.playbackSpeed = 2.0; // set the playback speed
});
Available Methods
Name | Description |
---|---|
pause() | To pause the playback |
play() | To start the playback after pausing it. |
rewind() | To jump to the start for the event playback. |
jumpForward() | To advance play time by one unit time: frameRate * playbackSpeed |
jumpBackward() | To seek backward by one unit time: frameRate * playbackSpeed |
reload() | To unmount and mount the player from scratch |
Available Getters
Name | Type | Description |
---|---|---|
tenantId | string | The Tenant ID. |
refreshToken | string | The refresh token from Tenant Login. |
accessToken | string | The access token from the Tenant Login. |
eventId | string | The Event ID. |
endpointId | number | The Endpoint ID. |
time | number | A timestamp in UNIX for Recording playback. |
hideWidgets | boolean | To hide the Player widgets. |
hideMap | boolean | To hide the Player map. |
hideHeader | boolean | To hide the header information with the Camera name. |
hideControls | boolean | To hide the player controls. |
hideTimeBrowser | boolean | To hide the day-hour-based timeline browser. |
hideBoundingBox | boolean | To hide the bounding boxes. |
playbackSpeed | number | To get the current playback speed. |
disableWakeUp | number | To disable automatic waking up from the parking mode |
hideSpeedWidget | boolean | To hide the speed widget from the video |
showEventList | boolean | To display a list of related events beside the player |
currentTime | number | To get the current player timestamp in UNIX |
forceStackedView | boolean | To retrieve the current status of the player's stack view mode. |
forceThumbnailView | boolean | To retrieve the current status of the player's thumbnail view mode. |
forceSplitView | boolean | To retrieve the current status of the player's split view mode. |
Available Setters
Name | Type | Description |
---|---|---|
tenantId | string | The Tenant ID. |
refreshToken | string | The refresh token from Tenant Login. |
accessToken | string | The access token from the Tenant Login. |
eventId | string | The Event ID. |
endpointId | number | The Endpoint ID. |
time | number | A timestamp in UNIX for Recording playback. |
hideWidgets | boolean | To hide the Player widgets. |
hideMap | boolean | To hide the Player map. |
hideHeader | boolean | To hide the header information with the Camera name. |
hideControls | boolean | To hide the player controls. |
hideTimeBrowser | boolean | To hide the day-hour-based timeline browser. |
hideBoundingBox | boolean | To hide the bounding boxes. |
playbackSpeed | number | To get the current playback speed. |
disableWakeUp | number | To disable automatic waking up from the parking mode |
hideSpeedWidget | boolean | To hide the speed widget from the video |
showEventList | boolean | To display a list of related events beside the player |
forceStackedView | boolean | To set the current status of the player's stack view mode. |
forceThumbnailView | boolean | To set the current status of the player's thumbnail view mode. |
forceSplitView | boolean | To set the current status of the player's split view mode. |
Available Events
Name | Description |
---|---|
load | It gets triggered after the data is loaded and ready to be played. |
event-click | When an event is clicked on the event list sidebar. The data property has the Event ID. |
camera-click | When Camera details page redirection is requested. The data property has the Endpoint ID. |
Sample Code
Download example from https://storage.googleapis.com/sai-web-libs/dashcam-player/example.zip
Extract the ZIP archive and open index.html
Live: https://storage.googleapis.com/sai-web-libs/dashcam-player/v4/index.html
Open the index.html file or the above link in your browser. You will be directed to the Login and Tenant selection pages. After completing authentication you will find a page like this:
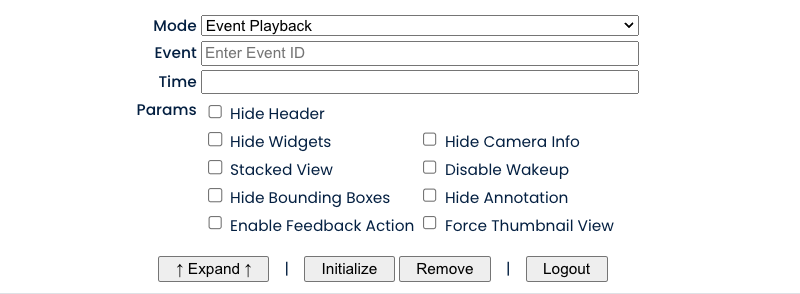
Select your playback mode, fill up the required input fields, and click Initialize Player to load the player.
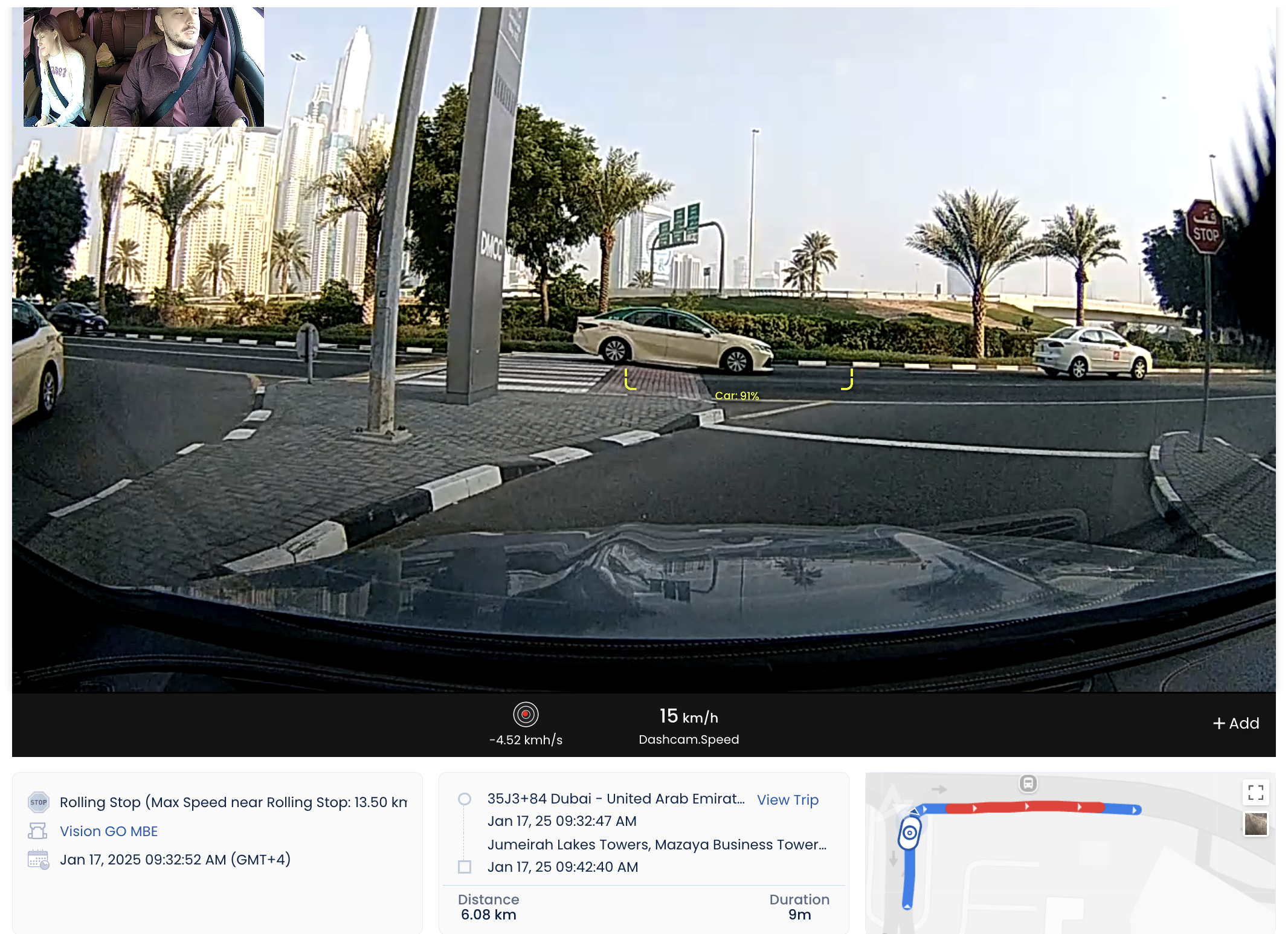
Changelog
2025.08.02
- Bug Fix: Handling video and sensor data download cancellation properly.
2025.07.11
- Feature: Persistent viewing mode.
- Feature: Meaningful naming for downloaded files and folders.
- Improvement: Enhanced video and frame extraction for split-view mode.
- Improvement: Optimized video decoding to reduce errors.
- Improvement: Revamped event timeline UI
- Improvement: Enhanced play/pause animation for smoother transitions.
- Bug Fix: Fixed bounding box issue for single image in split-view mode.
- Bug Fixes
2025.05.07
- Feature: Resize video thumbnails, collapse or expand them.
- Feature: New widget to visualize car movement.
- Feature: Trip information in the Event playback.
- Feature: Side-by-side split view.
- Feature: New video seek bar for Event player. Drag and push up for frame-by-frame view.
- Improvement: Grayscale map widget for better aesthetics.
- Improvement: Play/Pause using the spacebar.
2024.50.02
- Bug Fix: Hides imagers when no data is available.
2024.50.01
- Bug Fix - Fixed streaming issue
v2024.49.02
- Bug Fix - Bounding box font size bug
v2024.39.02
- Reports new property adjustment
- Bug Fixes
v2024.35.08
- Stream Duration and Paused Intervals are now part of the stream report.
- Bug Fixes
v2024.33.04
- Feature: Video downloads are restricted for the viewer role.
v2024.30.02
- Improvement: Video download reliability
v2024.26.11
- Improvement: When a video URL is not provided, the player will display "Video Unavailable."
- Bug fixes
v2024.12.08
- Improvement: Display when video decoding fails
- Bug fixes
v2024.08.03
- Improvement: Faster Event loading support (require to pass both refresh token and access token)
- Improvement: Updated Toast UI
- Improvement: Live-streaming stability
- Bug fixes
v2024.03.05
- Feature: Play events from SD-card
- Feature: New Bounding-box and Lane design
- Feature: Display 7 types of lanes
- Feature: Picture-in-picture map view over the video
- Feature: Audio recording playback
- Feature: Audio recording download
- Improvement: Select the main imager based on the trigger
- Improvement: Highlight the bounding box when the trigger was activated
- Improvement: Allow users to zoom in on the live-streaming map
- Bug fixes
v2023.43.03
- Feature: Exposed player stack view mode
- Bug Fix: No black screen for single imager
v2023.42.22
- Feature: Recent camera Events beside the player
- Feature: Movement and Speed widget on video
- Feature: Persistent user preference for player UI elements
- Improvement: Ability to open multiple player instances on the same page
- Bug fixes
v2023.40.14
- New getter:
currentTime
- New feature: Save the current view as PDF.
- New feature: Imperial or SI unit based on the device region
- Bug fixes
v2023.39.12
- New feature: Live streaming and edge recording requests will wake up the camera to complete the request
- New feature: Display a larger preview of the main image on the bigger screen.
- Improvement: The map displays stop signs for the Stop Sign Violation Events.
- Improvement: We have enhanced the precision of the most recent camera's location.
- Bug fixes
v2023.36.03
- New feature: Options to hide subcomponents.
- New feature: Methods to control play, pause, seek, rewind, and playback speed.
- New feature: Introduces a Timeline browser.
- Improvement: Updates sensor widgets design standards and automatically display related labels in the Events.
- Improvement: Revamp the player map view.
- Improvement: Redesign the player information area.
- Improvement: Adaptable player sizing for all screen sizes.
- Bug fixes.
v2023.32.02
- New feature: AI Labels mapping for bounding boxes.
- Improvement: Display stacked imagers on the small screen.
- Bug fixes for Live streaming.
v2023.27.02
- Initial version with basic capabilities for playing live streaming, recording, and events.